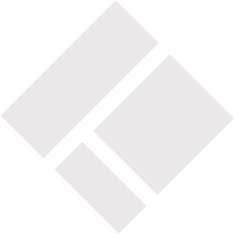
I have an app where I’m using Jbuilder to generate JSON for an API and I’m also using React. Sometimes, I want to pass the generated JSON in as props, but I need to nest it under the prop name. I found this not to be entirely straightforward.
I can easily get the rendered JSON string with the render
method and a Jbuilder partial by calling render 'path/to/partial.json', data: @data
, but if I want to nest that structure under another key, or manipulate it in any way I’m outta luck.
The solution I came up with was to add a helper method to ApplicationHelper
called from_jbuilder
. This returns a Ruby Hash / Array representation of the partial specified.
def from_jbuilder(template, options = {})
JbuilderTemplate
.new(self) { |json| json.partial! template, options }.attributes!
end
Now I can render the HTML for the component with:
<div
data-reat-class="MyComponent"
data-react-props="<%= { propName: from_jbuilder('path/to/partial.json', data: @data) }.to_json %>">
</div>